Why TypeScript?
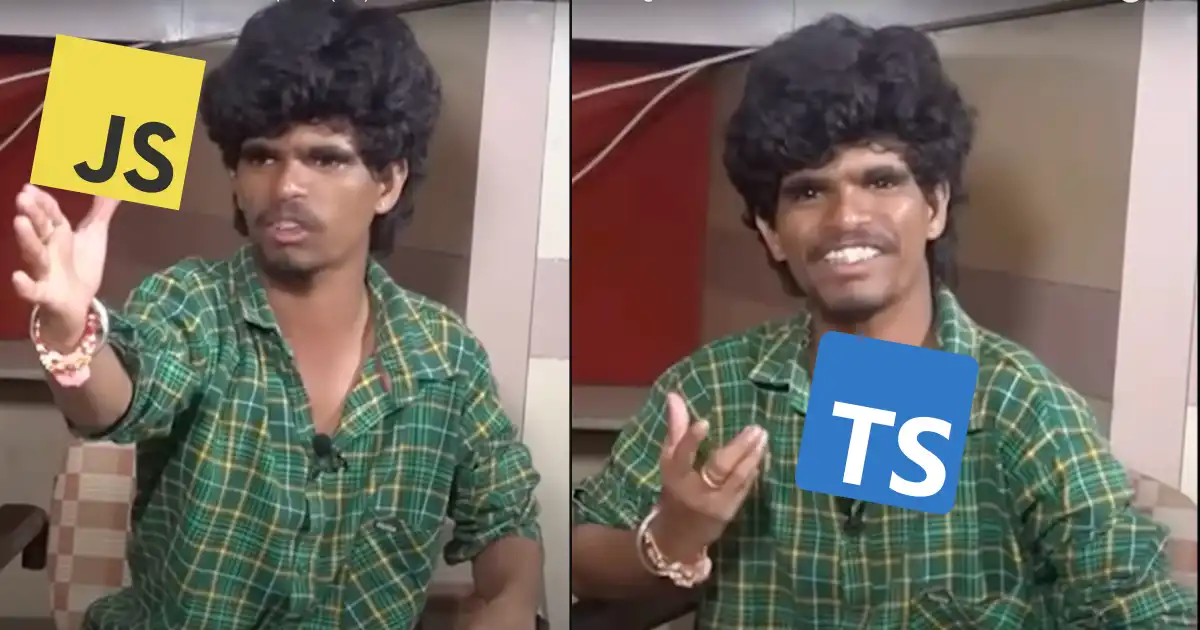
Hi everyone! 👋 if you’re in web-development world you would definitely heard about typescript!
Typescript is used to add data-types to your variables or functions!
ok 👍
- why do you need that?
- why not just use javascript?
Well basically javascript
is a dynamic language, which means you can assign any type of value to a variable.
// we declare a variable with number
let a = 10;
// then we can assign a string to it, it works but this might cause some issues!
a = "hello";
Typescript resolves this issue, here is example:
// we declare a variable with data-type number
let a: number = 10;
// then if we try to assign a string to it, we'll get an warning from our editor itself!
a = "hello"; // ❌ Type 'string' is not assignable to type 'number'.
Yeah i know shifting from Javascript
to Typescript
is a bit difficult, it will be annoying to write types for everything, but but! once you get a hang of it. It becomes drug 💊
Advantages of using Typescript
- Code becomes more predictive, you can skip simple data-type mistakes
const [number, setNumber] = useState<number>(0);
setNumber("hello"); // ❌ Type 'string' is not assignable to type 'SetStateAction<number>'.
- Handling complex data-structures becomes easier
type User = {
name: string;
sex: 'male' | 'female';
}
const users: User[] = []
// this will throw an error
users.push({
name: 'Uppal bal'
sex: null
})
- First class support for auto-suggestions by your editor
Type-safety without typescript?
JsDoc
You can use JsDoc
to add types to your javascript code, but it won’t be as good as typescript. here is an example:
/**
* @param {number} a
* @param {number} b
* @returns {number}
*/
function add(a, b) {
return a + b; // if you pass a string to it, you won't get any error
}
Bonus
End-to-end typesafe
If your full-stack frameworks like Next.js, Remix etc or using a Monorepo where frontend, backend code written in javascript
you can use these libraries and get type-safety between your frontend and backend code😘.
Validation
You can these libraries to validate your data-types on run-time
That’s it for this blog hope you enjoyed it! ✌️Peace