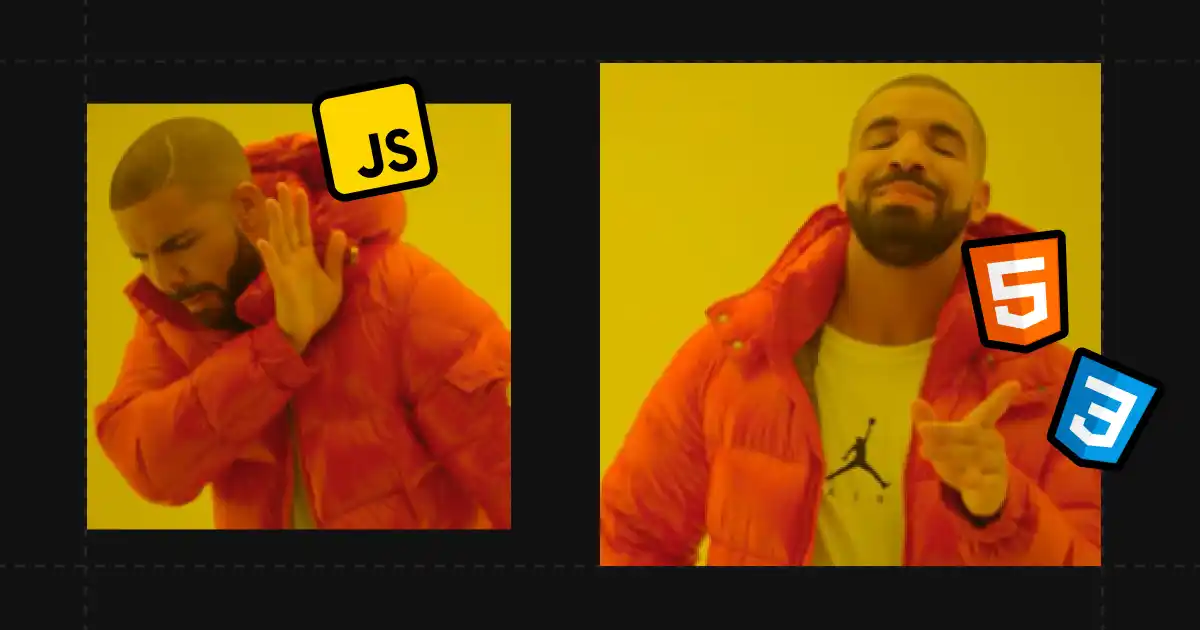
No Need for Javascript
# Javascript
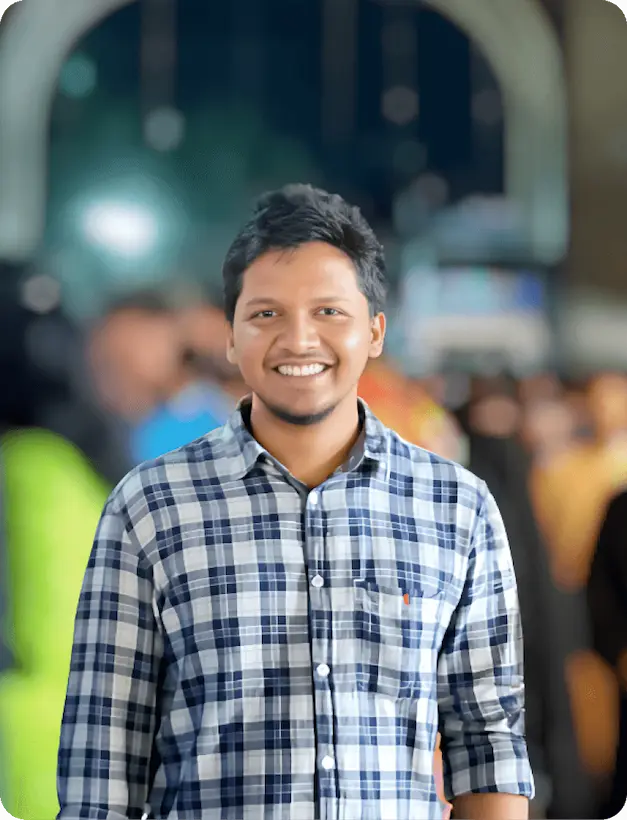
Pavan
Hey there 👋! Just finished washing my shoes 👟—they’ve been lying around for a while. Today, let’s dive into some things that can be done without relying on JavaScript.
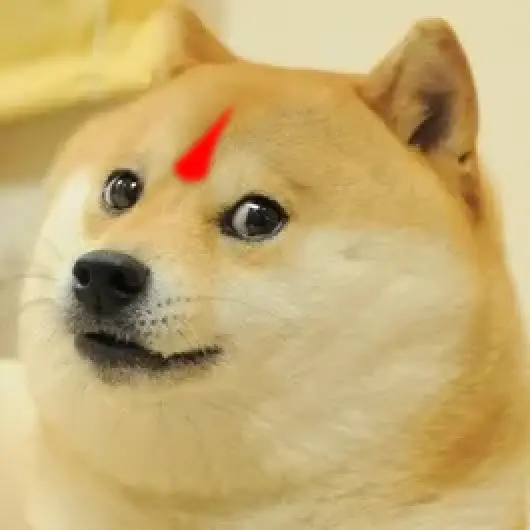
Chimtu
Aha! sounds interesting🙌
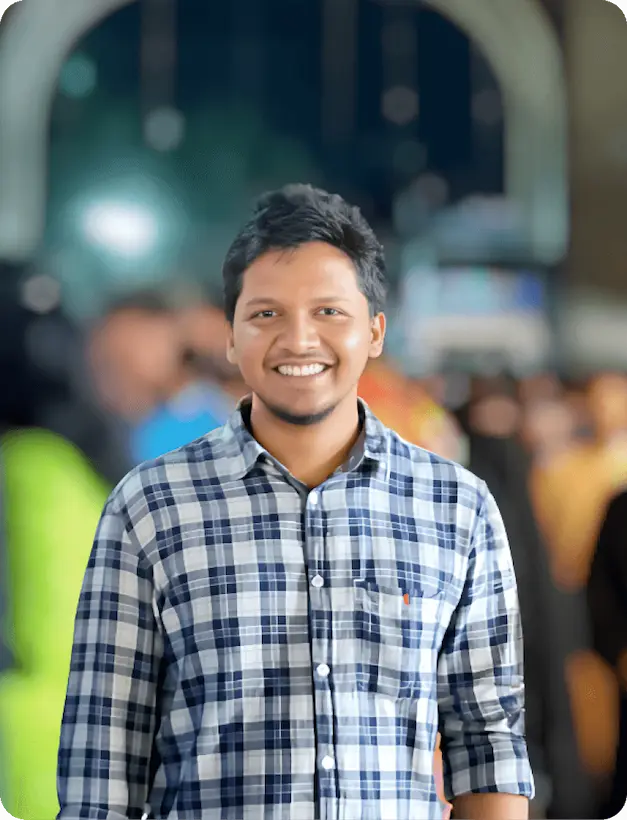
Pavan
Text Truncation…
- Text truncation is simple, yet it’s often overcomplicated. Here’s an example of a not-so-optimal approach
let sentence = "This is a string";
let result = sentence.slice(0, 4) + "...";
console.log(result); // result -> This...
- We can make text truncation with
CSS
& it’s responsive
/* This will add ellipsis for text in single line */
p {
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
- Want to truncate after 2 or 3 lines? Use this👇
p {
overflow: hidden;
-webkit-line-clamp: 2; /* Number of lines to show before truncating */
display: -webkit-box;
-webkit-box-orient: vertical;
}
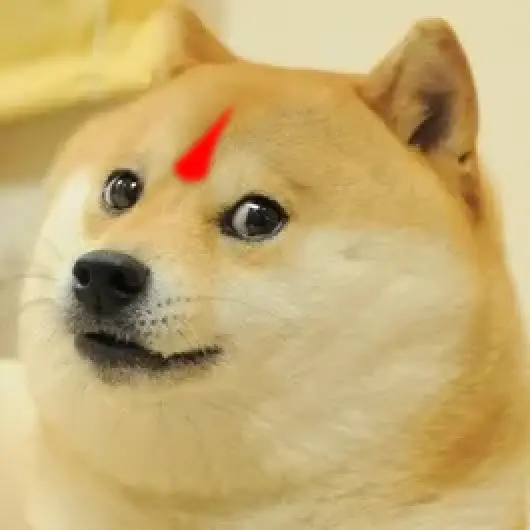
Chimtu
Oh, I know one! Scrolling!
Scrolling
- Scrolling to a particular section is often overdone with
ref
,getElementById
, and Javascript listeners - It can be done using the
<a>
tag, here is a example👇
<!-- Add smooth scrolling behavior -->
<head>
<style>
html {
scroll-behavior: smooth;
}
</style>
</head>
<!-- Add that id to the a tag -->
<nav>
<a href="/#pricing-section">Pricing</a>
</nav>
<!-- Give an unique to the container that needs to be focused -->
<div id="pricing-section">Pricing Section</div>
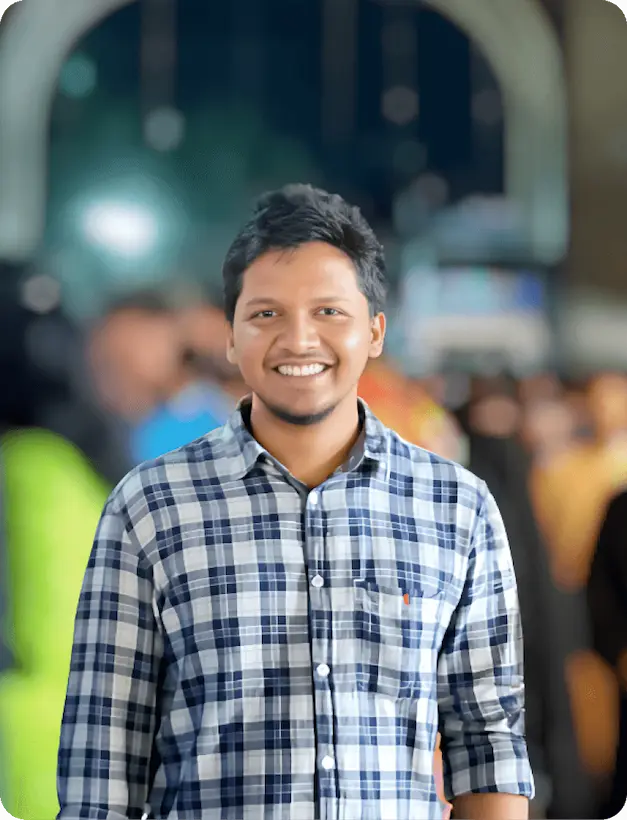
Pavan
Styling based on order
- Sometimes, you need to style elements differently based on their position. For instance, styling alternate rows in a table. Here’s a JavaScript-heavy approach
<ul>
{[1, 2, 3, 4, 5].map(i => (
<li key={i} style={{ color: i % 2 === 0 ? "gray" : "white" }}>
{i}
</li>
))}
</ul>
- We’ve CSS selectors to handle this stuff
<!-- :nth-child selector is used to select particular child -->
<!-- here i've selected even child -->
<head>
<style>
ul li:nth-child(even) {
color: red;
}
</style>
</head>
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
</ul>
- These are different css-selectors👇
/* even child style */
li:nth-child(even) {
color: red;
}
/* odd child style */
li:nth-child(odd) {
color: red;
}
/* particular child style */
li:nth-child(10) {
color: red;
}
/* styles first child */
li:first-child {
color: red;
}
/* styles last child */
li:last-child {
color: red;
}
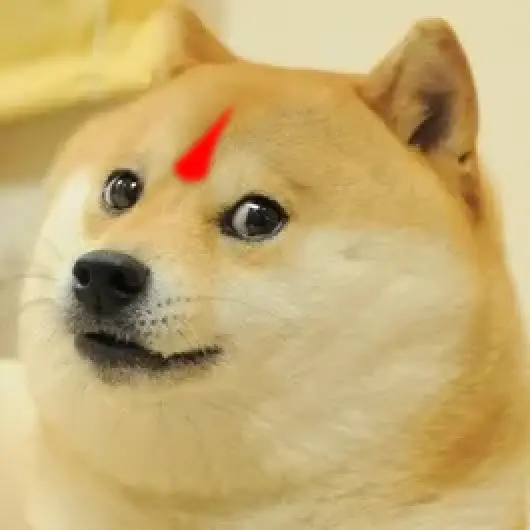
Chimtu
Shipping less javascript can speed up websites, mean better performance
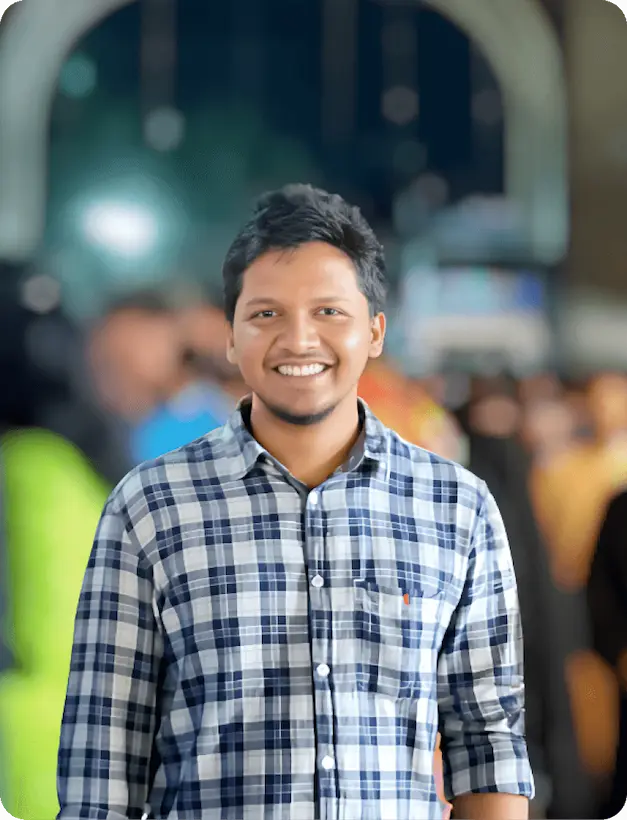
Pavan
Yeah that’s true! thanks for making till here, see you in next blog! peace✌️